Web Scraping with Cheerio and Node.js: A Comprehensive Guide
Scraping static web pages can be challenging, but Cheerio makes it fast and efficient. Cheerio is a lightweight Node.js library that parses and manipulates HTML using a syntax similar to jQuery. This guide covers key concepts, practical code examples, and essential techniques to help you extract web data with ease—no matter your experience level.
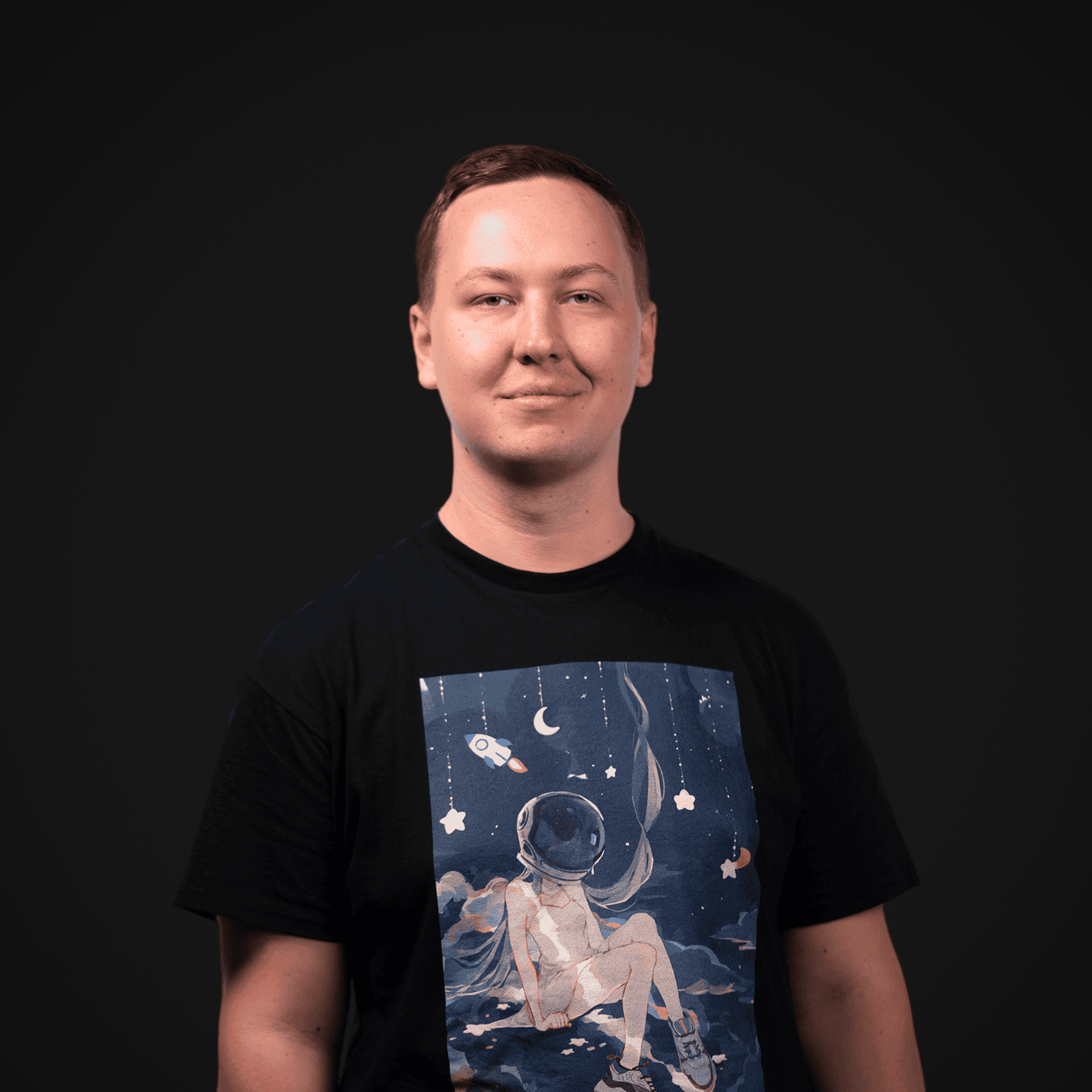
Zilvinas Tamulis
Mar 04, 2025
6 min read
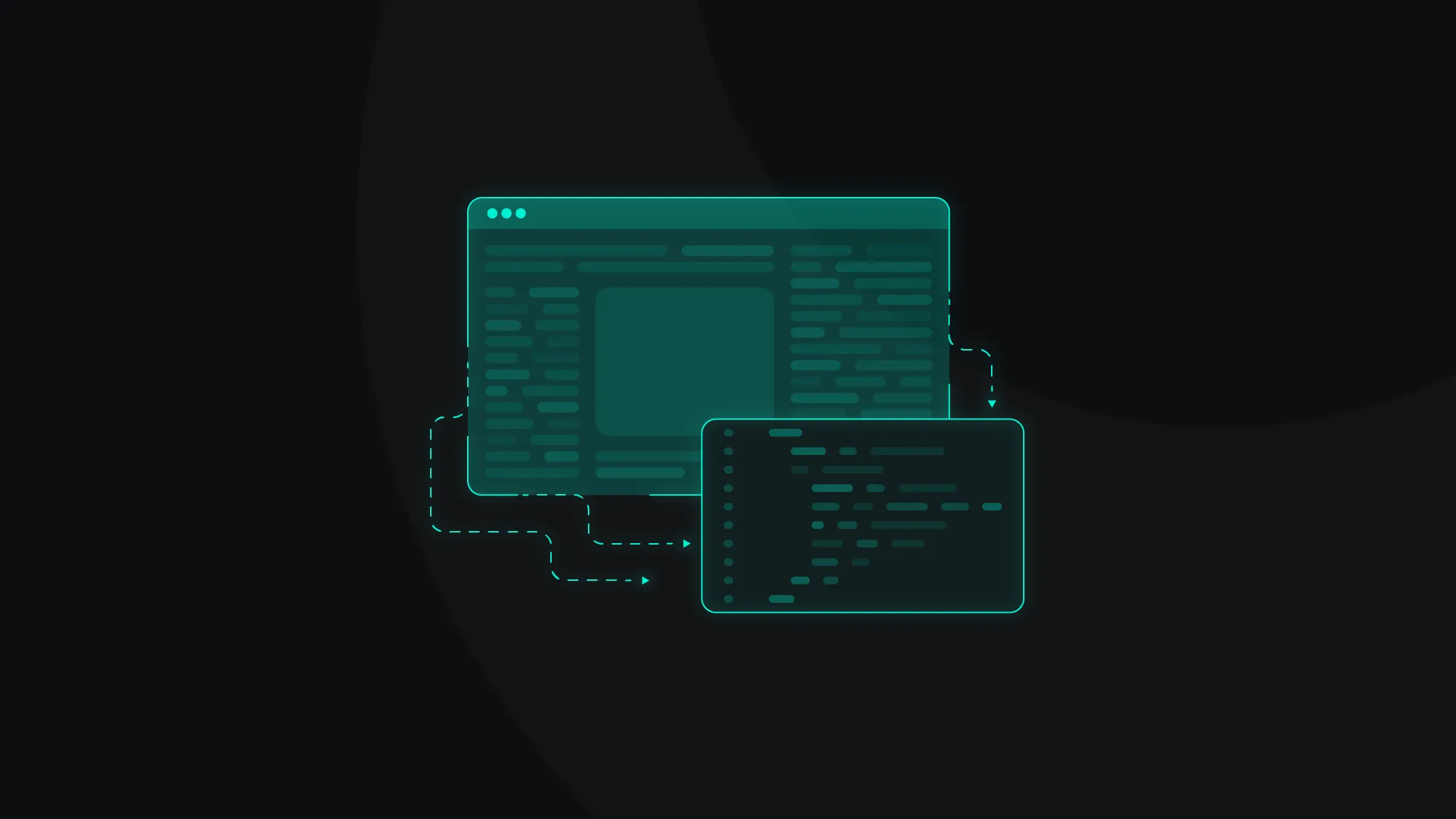
What is Web Scraping?
Web scraping is a fundamental technique for extracting structured data from websites. Understanding the basics of web scraping is crucial for conducting market research or gathering content for analysis.
Web scraping is the process of automatically extracting data from web pages. Businesses and developers use it for:
- Market research – gathering competitor pricing, customer sentiment, and trends.
- SEO monitoring – analyzing search engine rankings and keyword usage.
- Content aggregation – collecting and organizing information from multiple sources.
- Data analysis – extracting insights from public datasets.
Web scraping comes with challenges such as legal considerations, CAPTCHA protection, and anti-bot mechanisms. Ethical scraping involves respecting a site's robots.txt file and ensuring responsible data collection.
Why Choose Cheerio for Web Scraping?
Before diving into practical examples, it's essential to understand why Cheerio is a popular choice for web scraping in Node.js projects.
Cheerio is a lightweight library for server-side HTML parsing. It offers a jQuery-like syntax that makes traversing and manipulating the DOM intuitive.
Tools explained:
- jQuery – a JavaScript library that makes it easier to use JavaScript on your website. It helps with tasks like finding and changing parts of a webpage, handling events like clicks, creating animations, and working with data without reloading the page.
- htmlparser2 – a tool for Node.js (a JavaScript runtime) that reads and understands HTML and XML documents. It works by building a tree of elements, similar to how browsers understand webpages.
jQuery simplifies HTML document manipulation in the browser. Under the hood, Cheerio uses htmlparser2, a fast and forgiving HTML parser that converts raw HTML into a traversable DOM structure. However, you don't need to worry about the complexities of htmlparser2, as Cheerio abstracts those details away.
Key advantages of Cheerio
- Speed & efficiency – with no browser overhead, Cheerio is significantly faster than headless browsers.
- Lightweight – consumes minimal resources, making it ideal for small-scale tasks.
- jQuery-like Syntax – Cheerio offers an easy-to-use interface for DOM traversal and manipulation for developers familiar with jQuery.
- Great for static pages – perfect for scraping HTML content that does not require JavaScript rendering.
When NOT to use Cheerio
For pages that load content dynamically via JavaScript, consider tools like Playwright or Puppeteer that simulate a complete browser environment.
Now you understand key Cheerio's advantages for scraping static web pages. This knowledge will lay a solid foundation for the practical examples that follow.
Setting up the environment
Getting started with Cheerio requires a basic Node.js setup. This section will cover the prerequisites and installation steps to begin your scraping project.
Node.js installation
Go to the official Node.js page and download the latest version. When downloaded, follow the installation steps.
Create a project
Open the terminal and initialize the project in the target folder:
After initialization, the package.json file will be created.
Packages installation
Install Cheerio and Axios:
Axios makes HTTP requests to fetch web pages, a crucial step in the web scraping process, and Cheerio parses and manipulates the HTML content.
We've set up the required environment by installing Node.js, Axios, and Cheerio, ensuring you have the tools for efficient web scraping.
Example: scraping web pages with Cheerio
Let’s use a practical example to extract product titles and prices from an e-commerce website. Each code snippet is explained in detail to ensure you understand every process step.
Step 1: fetching the web page content
Axios is used to fetch the HTML content from the given URL. If the request succeeds, the HTML is returned; if it fails, an error message is logged. This raw HTML serves as the basis for data extraction.
Step 2: parsing the HTML and extracting data
After fetching the HTML, we load it into Cheerio, which lets us use familiar jQuery-like selectors. The code then loops through each element with the class product-item, extracts the title and price, and logs an array of product objects.
Advanced techniques
Once you have the basics down, more complex scenarios may arise. Let’s consider advanced techniques such as pagination, dynamic content handling, and robust error management.
Handling pagination
This function demonstrates how to iterate through multiple pages by dynamically constructing page URLs. It calls the scrapeData function for each page, ensuring you can seamlessly scrape a series of pages.
Dealing with JavaScript-rendered content
Cheerio is insufficient for websites that load data via JavaScript. This example uses Playwright to launch a headless browser, navigate to the target page, and retrieve the fully rendered HTML content.
Error handling
Robust error handling is crucial. This function uses a try-catch block with a timeout option to manage connection failures and timeouts, returning null if an error occurs.
This section has expanded on advanced scraping techniques, including pagination, dynamic content handling, and comprehensive error management, ensuring you're well-equipped for more challenging projects.
Debugging and optimization tips
Effective debugging and optimization are crucial for building a robust, efficient web scraper. This section provides in-depth strategies for diagnosing issues, monitoring performance, and fine-tuning your code.
Best debugging tips you can follow:
- Use developer tools – use Chrome DevTools (or similar browser tools) to inspect the DOM structure of your target pages. This will help you verify that your selectors correctly identify the desired elements.
- Log intermediate data – insert console.log statements or use a logging library to print intermediate outputs. This helps track the data flow through your scraper and pinpoint where unexpected values or errors occur.
- Utilize Node.js debugging tools – you can examine your code using Node.js’s built-in debugger or third-party tools like node-inspector to identify performance bottlenecks or logic errors.
- Error handling and reporting – implement comprehensive try-catch blocks around critical sections of your code. Log errors systematically and consider writing them to a file or alerting you when issues arise.
- Unit testing – write unit tests for core functions using frameworks like Mocha or Jest to ensure each component behaves as expected.
Performance optimization
To ensure that you're accessing the data you need without any interruptions, you must constantly optimize your workflows. Here are a few expert tips:
- Optimize selectors – refine your CSS selectors to target only the necessary elements, reducing DOM traversal time. Testing selectors in the browser console can help ensure efficiency.
- Reduce redundant requests – limit HTTP requests by caching repeated requests when possible. This prevents unnecessary network calls and speeds up the scraping process.
- Leverage asynchronous processing – use Node.js’s asynchronous capabilities (async/await or Promises) to handle concurrent requests efficiently without overwhelming your resources.
- Monitor resource usage – utilize performance monitoring tools to track memory, CPU, and network usage. Tools like console.time() can help measure operation durations and identify slow segments of your code.
- Implement rate limiting – insert delays between requests using randomized intervals to mimic natural browsing behavior and avoid IP bans.
- Code profiling – use Node.js profiling tools to analyze which parts of your code are resource-intensive, guiding you where to focus optimization efforts.
With these detailed debugging and optimization strategies – from utilizing developer and Node.js tools to refining selectors and implementing rate limiting, you can build a web scraper that is both resilient to even the most advanced anti-bot mechanisms and is efficient, even under high loads.
Best practices for web scraping with Cheerio and Node.js
Following best practices is essential for ensuring that your web scraping projects are practical and responsible. This section provides detailed guidelines on technical, ethical, and operational practices to help you build robust and maintainable scrapers.
Respect website policies
- Adhere to robots.txt – always check the target website’s robots.txt file to understand which parts of the site can be scraped.
- Review Terms of Service (ToS) – ensure your scraping does not violate the website’s ToS.
- Data privacy and copyright – only scrape publicly available data and consider anonymizing sensitive information.
Optimize your scraping strategy
- Efficient selectors – use precise CSS selectors to target only the necessary elements.
- Rate limiting and throttling – implement delays between requests to mimic natural behavior and avoid IP bans.
- Proxy management – leverage rotating proxies to avoid CAPTCHAs, IP blocks, and other restrictions while collecting data from advanced targets.
- Asynchronous processing – utilize Node.js’s async capabilities to handle concurrent requests without overloading resources.
Check the quality of your data
- Data validation and cleaning – clean and validate data using Regular Expressions or libraries such as Lodash.
- Error handling – use try-catch blocks and log errors systematically.
- Unit testing and monitoring – write unit tests and continuously monitor your scraper for changes in website structure.
Maintain and regularly update your scraper
- Monitor changes – regularly check for updates in website structure and update your code accordingly.
- Documentation and code comments – maintain clear documentation and inline comments for future updates.
- Scalability – consider distributed architectures using message queues or serverless functions for large-scale projects.
Following these best practices ensures that your web scraping projects are technically sound, legally compliant, and ethically responsible. They also maintain high data quality and adaptability to future changes.
Bottom line
Cheerio is a powerful, lightweight tool for efficiently extracting data from static web pages. By following the detailed examples, advanced techniques, and best practices presented in this guide, you can build robust scrapers that are both efficient and ethical. For JavaScript-heavy sites, consider integrating tools like Playwright or Puppeteer.
This guide has equipped you with a comprehensive understanding of web scraping with Cheerio, enabling you to tackle various data extraction challenges confidently.
Test residential proxies with #1 IP quality in the market
Activate your 3-day free trial with 100MB and enhance your scraping projects with 125M+ IPs from 195+ worldwide locations.
About the author
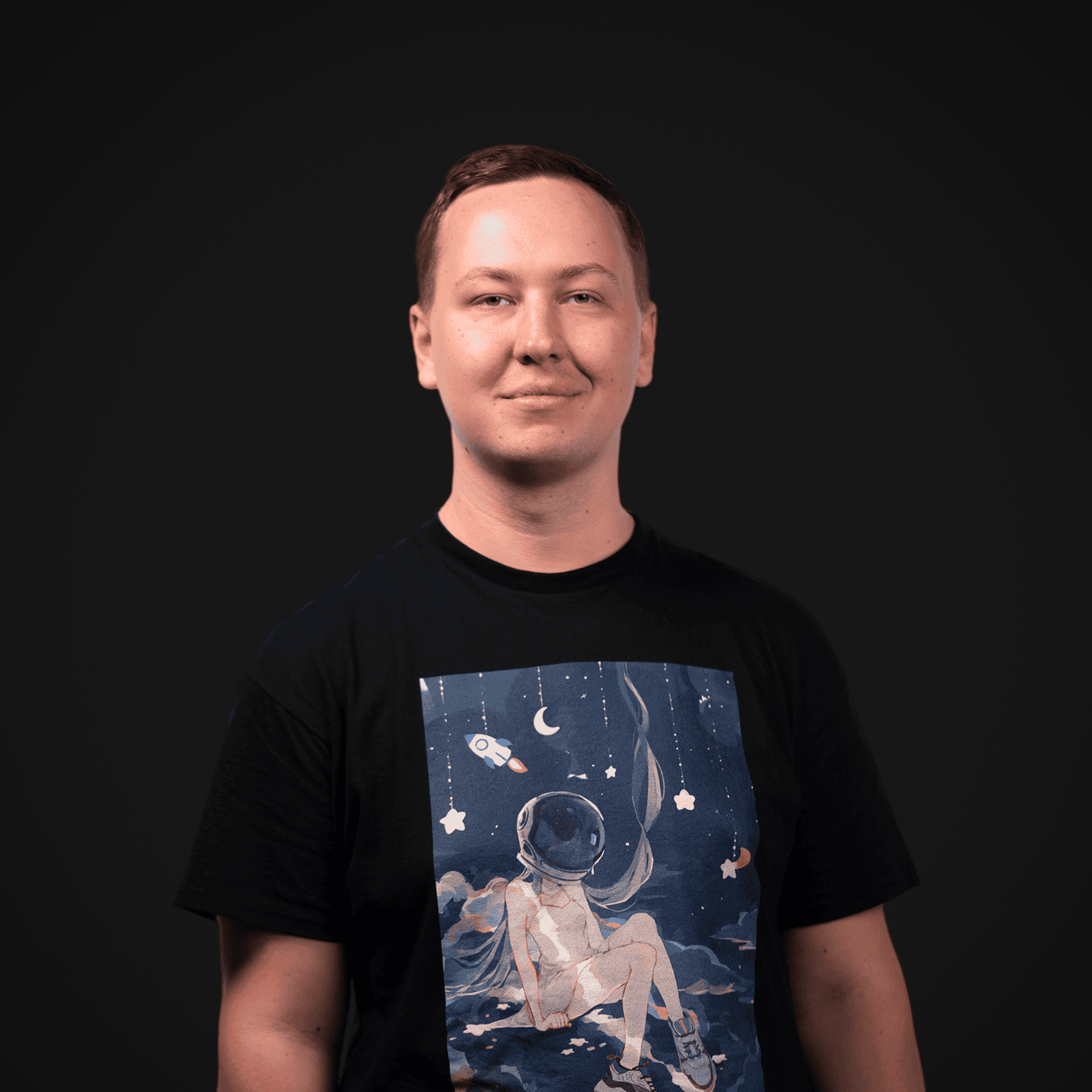
Zilvinas Tamulis
Technical Copywriter
A technical writer with over 4 years of experience, Žilvinas blends his studies in Multimedia & Computer Design with practical expertise in creating user manuals, guides, and technical documentation. His work includes developing web projects used by hundreds daily, drawing from hands-on experience with JavaScript, PHP, and Python.
Connect with Žilvinas via LinkedIn
All information on Decodo Blog is provided on an as is basis and for informational purposes only. We make no representation and disclaim all liability with respect to your use of any information contained on Decodo Blog or any third-party websites that may belinked therein.